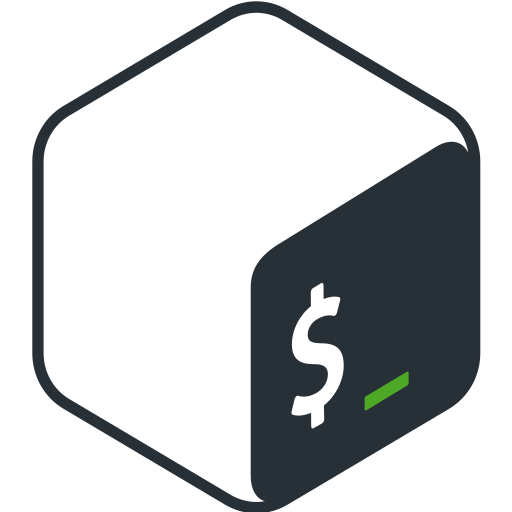
The biggest key to being a great Linux Systems Administrator is your ability to write Bash scripts. Bash scripts recover days of our time by automating all the day-to-day operations on our servers. However, writing a script, and writing a secure bash script are two different things.
Your code may work well under normal operation, but an attacker may be able to leverage it against you. So how can we ensure our scripts are not used against us or behave unexpectedly?
Avoid the Bash Pitfalls
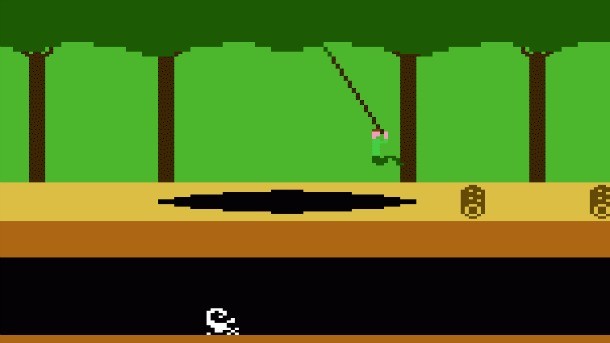
Write good code! Seems simple, right? Well, there are a lot of bash programming pitfalls you need to avoid. Bash is one of those languages that are really good at working with best-guess estimates. So we need to first be aware that just because our script works does not mean it’s the correct way to write it.
There are so many pitfalls in bash that I could not list them all here. Luckily there is already a great resource of Bash programming “Do’s and Don’ts”.
My go-to guide is Greg’s Wiki. Read through all the examples and then review your own code again. There will very likely be some lines you will want to rewrite.
URL: http://mywiki.wooledge.org/BashPitfalls
Use Exit Codes
Every individual command that runs has its own exit status code. The command’s exit code number will tell you if a command ran successfully or failed. To ensure key commands in your scripts execute correctly you should add in checks on the commands exit codes.
In any Bash script or at the command line you can see the exit code of the last command by running a simple “echo $?” command. If the resulting output is anything other than “0” then the command failed.
Let’s put this all together in a script that will restart the SSH service and confirm the service restart is successful.
# Declare variable
declare str_cmd='systemctl restart ssh.service'
# Run the command
/bin/bash <<<"""${str_cmd}"""
# Check the commands exit-code status.
if [[ "$?" == 0 ]] ; then
echo 'Command Exit Code SUCCESS'
else
echo 'Command Exit Code FAIL!'
fi
# P.S. - I know there is a better way to write this "if" test,
# but this version makes the concept easier to follow.
Adding exit code checks to our scripts ensures it operates as you intend it to, and if it does not we can tell the script what to do when it fails. The goal is to always control the success or failure of the scripts commands.
More Bash Exit Code Resources
Static Bash Code Analysis
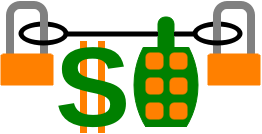
Static code analysis is great at pointing out obvious holes in your script. The code analysis tool will ingest a script and output the issues it discovers within the code that needs to be fixed. Each programming language will require its own static code analysis tool for that language.
Shellharden is a project dedicated to code analysis of Bash scripts. The tool mainly focuses on the correct use of quotations within a script. The misuse of quotations or the lack of their use can leave a script open for abuse. So fixing quotation vulnerabilities is a central part of keeping your script safe.
Follow the link below to get started analyzing your code.
URL: https://github.com/anordal/shellharden