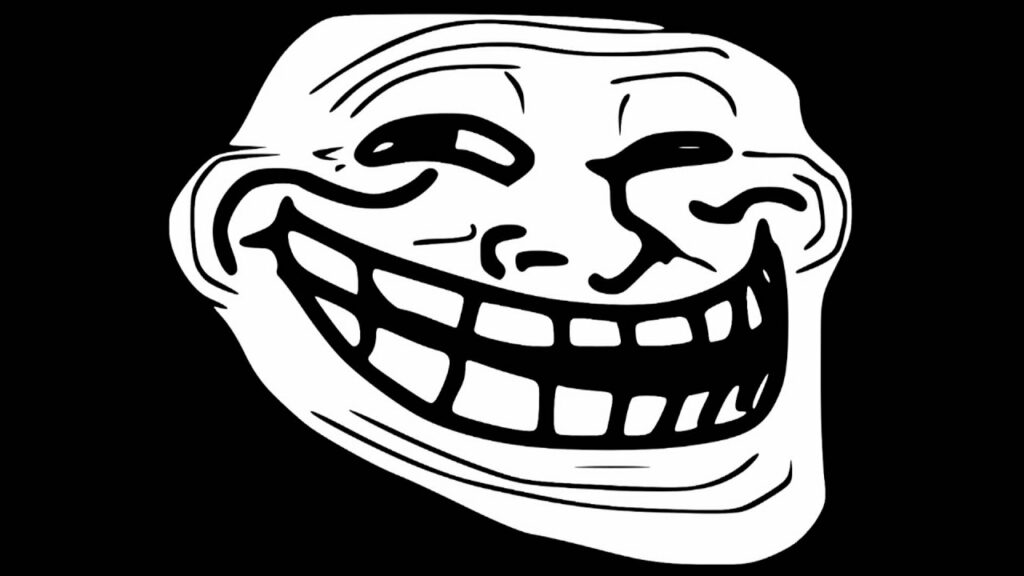
Man do I hate spammers! Phishing spam is typically unsophisticated yet, unfortunately, highly effective. Managing email phishing risks require constant user training and time. It is a constant annoyance. Plus, phishing emails that trick users into giving up their Outlook passwords is a script-kiddie attack method. The Emotet spam at least had powerful and interesting malware to analyze; these other attacks are boring by comparison. So when I got an “Outlook password reset” phishing email in my inbox I decided to have some fun with the spammer.
The Incident Response
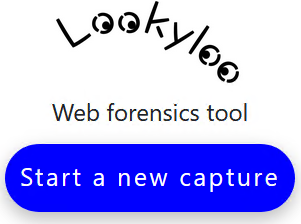
It started with the normal incident response, check the email’s source, extract URLs, and run the URL in a sandbox. I use a great online tool called LookyLoo. Lookyloo is an online tool that allows you to quickly and safely follow malicious URLs. Most email malware will send you through a bunch of 302 redirects to “launder” the malicious URL. LookyLoo makes this really easy to follow all the 302s. Side note: Burpsuite is also great for following “launder” malicious URLs.
Here is what LookyLoo found.
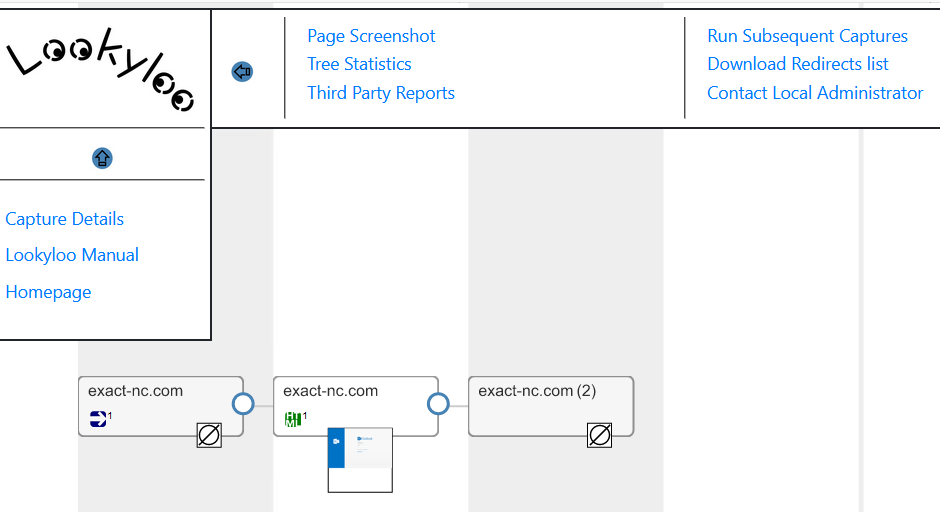
LookyLoo found only one redirect and once at the URLs final destination took a image of the webpage. Below is the image of that page.
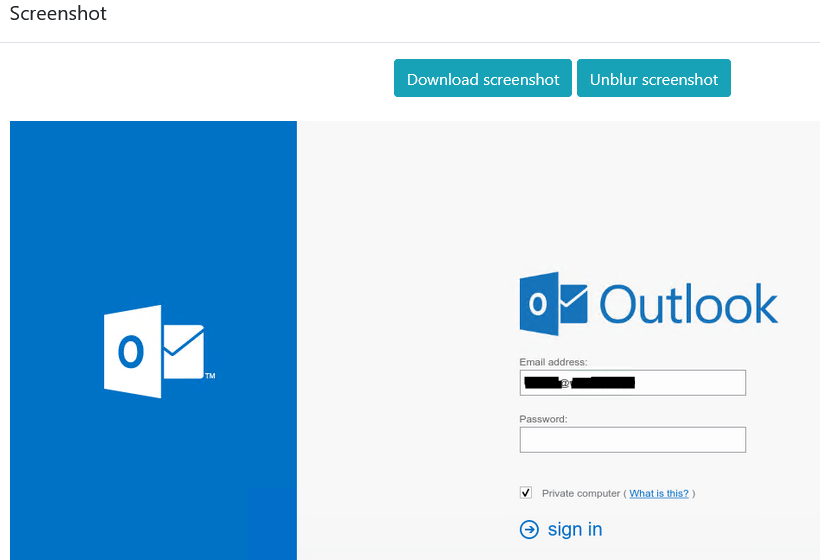
Analysis
After reviewing all the details in the LookyLoo report, I confirmed the webpage was just a phishing attack. There was no drive-by-download malware in the web contents. Now that I know the page is safe to browse to I will open it in a sandbox VM. After getting the URL opened up, I filled in the fake Outlook login with bogus data a few times while keeping Firefox’s persistent networking log on. Reviewing the Firefox networking logs, revealed the simple POST request made by the webpage when submitting data.
It was at this point I put on my Gray Hat…
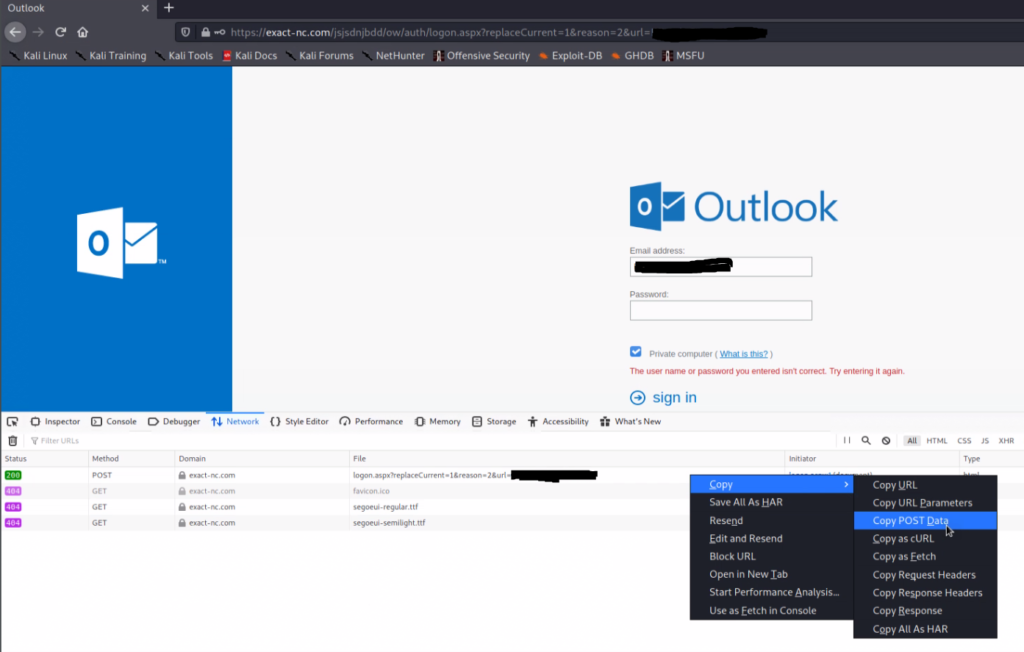
That Disclaimer…
This last part I don’t recommend you do and is for educational purposes only. While this was clearly an illegal phishing website sent up to steal credentials, sending it bogus data is definitely a legal gray area. However, the spammer did ask me for my input on his phishing webpage. So I am simply obliging his request.
Gray Hat Time
What could I do to disrupt this phishing attack? Spam campaigns are a spray and pray type of attack normally. The spam could have been sent to a lot of domains. I could easily spam the spammers phishing page. Maybe the spammer even set up email alerts for when he got a hit on the phishing page? That could be fun!
I started with a simple “while true” bash loop, sending the same email address and password. The password vulgarly and explicitly told the spammer what I thought about him. I let that run for a few minutes until I realized, that would not disrupt the spammer’s campaign. The spammer could easily filter out all my POSTs from his data. This would leave Users who were tricked into giving up their password at risk, and we just cannot have that.
Needs To Be Random
To make the spammer’s data useless we need to randomize every detail about our HTTP POSTs requests. There are four variables we need to randomize; email address, password, the browser’s User-Agent, and our IP address.
To randomize the email address, password, and browser’s User-Agent data I quickly found resources on GitHub.
- UserName: https://github.com/danielmiessler/SecLists/blob/master/Usernames/Names/names.txt
- Passwords: https://github.com/danielmiessler/SecLists/tree/master/Passwords
- DomainNames: https://github.com/opendns/public-domain-lists
- User-Agents: https://github.com/tamimibrahim17/List-of-user-agents
The Domain Names and User-Agents lists each have multiple lists. For each I combined them into one list and sorted out duplicates. Now I have one large list of Domain Names and one large list of User-Agents. All this data is real data, not random gibberish.
We will use TOR to randomize the our IP address. To do this we will set up TOR as a SOCKS proxy and configure it to change its IP frequently, after every request sent. Then we just tell cURL to send our POSTs through our local TOR SOCKS proxy.
# TOR Set up
# Install
apt update && apt install tor
# Config to Us US based ExitNodes only, and change the IP after every request.
echo "StrictNodes 0
ExitNodes {us}
MaxCircuitDirtiness 1" >> /etc/tor/torrc
# Restart TOR and check the status.
systemctl restart tor && systemctl status tor
Quick and Dirty Bash
A quick and dirty Bash script can handle this job for me. The script takes in four lists of data. A random line is selected from the UserName List and the Domain Name list to create a new random email address. A random line from the Password list is selected for a password and the same is done for the User-Agent. The “DATA” field of the POST data has to be URL encoded to look legit, so I added a function to do that. The random data is plugged into the cURL command and sent off via the TOR SOCKS proxy. There are two cURL commands to match the exact input process the spammer set up. Again, we need to make it look as real as possible. After a round of POSTs is sent there is a random sleep timer, and then the whole process starts again.
#!/bin/bash
# Name: Spam_Lolz.sh
# List Variables
my_name="${0}"
un_list="${1}"
pw_list="${2}"
dn_list="${3}"
ua_list="${4}"
#
# Check all variables given
if [ -z "${un_list}" ] || [ -z "${pw_list}" ] || [ -z "${dn_list}" ] || [ -z "${ua_list}" ];then
echo "Error: Missing argument(s)."
echo "./${my_name} username-db.txt password-db.txt domainname-db.txt useragent-db.txt"
exit 1
fi
#
# Clear old Log
echo '' > ./Spam_loz.log
# URL Encoding Fuction
urlencode() {
old_lc_collate=$LC_COLLATE
LC_COLLATE=C
local length="${#1}"
for (( i = 0; i < length; i++ )); do
local c="${1:$i:1}"
case $c in
[a-zA-Z0-9.~_-]) printf '%s' "$c" ;;
*) printf '%%%02X' "'$c" ;;
esac
done
LC_COLLATE=$old_lc_collate
}
#
# Main While Loop
while true; do
pw="$(shuf -n 1 ${pw_list})"
email="$(shuf -n 1 ${un_list})@$(shuf -n 1 ${dn_list})"
ua="$(shuf -n 1 ${ua_list})"
# Output
echo "${email}"
echo "${pw}"
echo "${ua}"
echo '+++++++++++++++++++++++++++++++++++++++++++++++++++'
#
email_ec="$(urlencode "${email}")"
pw_ec="$(urlencode "${pw}")"
url="https://exact-nc.com/jsjsdnjbdd/ow/auth/logon.aspx?replaceCurrent=1&reason=2&url=${email}"
#
log=$(curl --socks5-hostname localhost:9050 -s 'https://exact-nc.com/jsjsdnjbdd/ow/auth/logon.aspx' -H "User-Agent: ${ua}" -H 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H 'Accept-Language: en-US,en;q=0.5' --compressed -H 'Content-Type: application/x-www-form-urlencoded' -H 'Origin: https://exact-nc.com' -H 'DNT: 1' -H 'Connection: keep-alive' -H 'Referer: https://exact-nc.com/jsjsdnjbdd/ow/auth/logon.aspx' -H 'Upgrade-Insecure-Requests: 1' --data-raw "username=${email_ec}&password=${pw_ec}&passwordText=&trusted=4&isUtf8=1")
#
echo ${log} >> ./Spam_loz.log
#
log=$(curl --socks5-hostname localhost:9050 -s "${url}" -H "User-Agent: ${ua}" -H 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' -H 'Accept-Language: en-US,en;q=0.5' --compressed -H 'Content-Type: application/x-www-form-urlencoded' -H 'Origin: https://exact-nc.com' -H 'DNT: 1' -H 'Connection: keep-alive' -H "Referer: ${url}" -H 'Upgrade-Insecure-Requests: 1' --data-raw "username=${email_ec}&password=${pw_ec}&passwordText=&trusted=4&isUtf8=1")
echo ${log} >> ./Spam_loz.log
unset log
# Sleep from 1 to 15 seconds randomly.
sleep $((1 + $RANDOM % 15))
done
exit 0
The Results
Oh I do enjoy this..
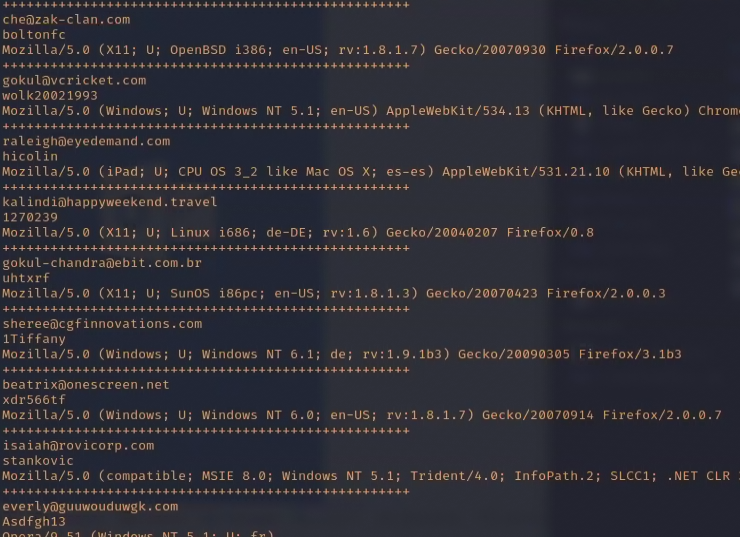
Leave a Reply